1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
|
# prettydiff
[](https://crates.io/crates/prettydiff)
[](https://docs.rs/prettydiff)
Side-by-side diff for two files in Rust. App && Library.
## Examples
### Slice diff
```rust
use prettydiff::diff_slice;
println!("Diff: {}", diff_slice(&[1, 2, 3, 4, 5, 6], &[2, 3, 5, 7]));
println!(
"Diff: {}",
diff_slice(&["q", "a", "b", "x", "c", "d"], &["a", "b", "y", "c", "d", "f"])
);
println!(
"Diff: {}",
diff_slice(&["a", "c", "d", "b"], &["a", "e", "b"])
);
```
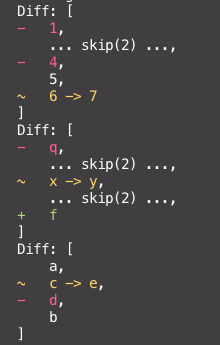
Get vector of changes:
```rust
use prettydiff::diff_slice;
assert_eq!(
diff_slice(&["q", "a", "b", "x", "c", "d"], &["a", "b", "y", "c", "d", "f"]).diff,
vec![
DiffOp::Remove(&["q"]),
DiffOp::Equal(&["a", "b"]),
DiffOp::Replace(&["x"], &["y"]),
DiffOp::Equal(&["c", "d"]),
DiffOp::Insert(&["f"]),
]
);
```
### Diff line by chars or words

```rust
use prettydiff::{diff_chars, diff_words};
println!("diff_chars: {}", diff_chars("abefcd", "zadqwc"));
println!(
"diff_chars: {}",
diff_chars(
"The quick brown fox jumps over the lazy dog",
"The quick brown dog leaps over the lazy cat"
)
);
println!(
"diff_chars: {}",
diff_chars(
"The red brown fox jumped over the rolling log",
"The brown spotted fox leaped over the rolling log"
)
);
println!(
"diff_chars: {}",
diff_chars(
"The red brown fox jumped over the rolling log",
"The brown spotted fox leaped over the rolling log"
)
.set_highlight_whitespace(true)
);
println!(
"diff_words: {}",
diff_words(
"The red brown fox jumped over the rolling log",
"The brown spotted fox leaped over the rolling log"
)
);
println!(
"diff_words: {}",
diff_words(
"The quick brown fox jumps over the lazy dog",
"The quick, brown dog leaps over the lazy cat"
)
);
```
### Diff lines
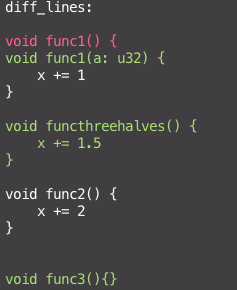
```rust
use prettydiff::diff_lines;
let code1_a = r#"
void func1() {
x += 1
}
void func2() {
x += 2
}
"#;
let code1_b = r#"
void func1(a: u32) {
x += 1
}
void functhreehalves() {
x += 1.5
}
void func2() {
x += 2
}
void func3(){}
"#;
println!("diff_lines:");
println!("{}", diff_lines(code1_a, code1_b));
```
## App
This crate also provides app for side-by-side diff:
```sh
cargo install prettydiff
prettydiff left_file.txt right_file.txt
```
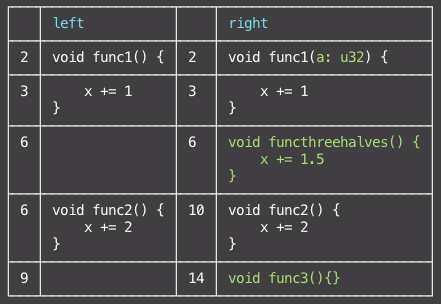
|